Okay, so, yesterday I tackled something kinda interesting: setting up a basic Jackson MS environment in about an hour. Sounds crazy, right? But hear me out.
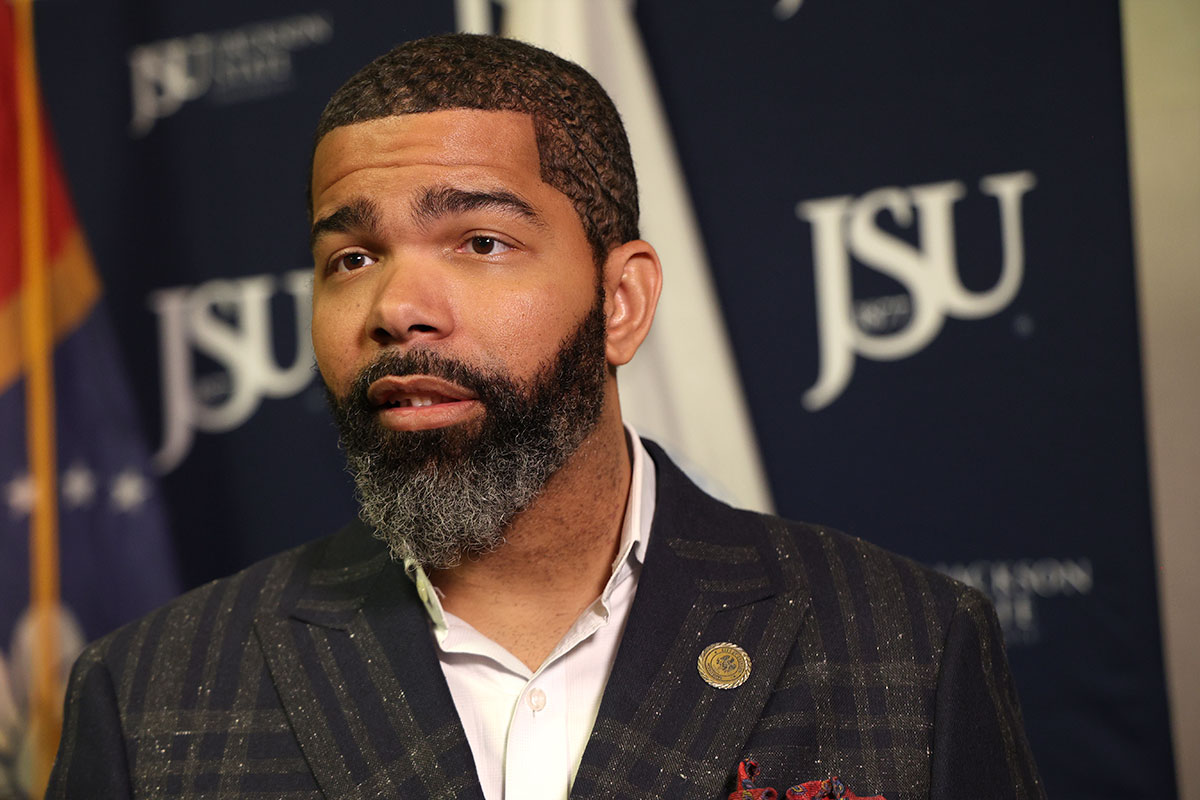
Started with the Prep Work
First off, I knew I couldn’t just dive in headfirst. I spent like, maybe 5 minutes just figuring out what I really needed. I was aiming for bare minimum: a functional setup for some basic JSON parsing and maybe some object mapping. No fancy stuff.
Grabbed the Essentials
Then, it was all about getting the right libraries. Headed over to Maven Central, searched for “Jackson core,” “Jackson databind,” and “Jackson annotations.” Downloaded the JARs. Could’ve used Maven or Gradle, but for a quick test, just grabbing the JARs felt faster, you know?
Set Up the Project (Quick and Dirty)
Created a simple Java project in IntelliJ (you can use Eclipse or whatever, doesn’t really matter). Made a new folder called “lib” and tossed all those Jackson JARs in there. Then, I added them to the project’s classpath. This took maybe 10 minutes, tops.
Wrote Some Test Code
Now for the fun part! I created a simple Java class with a `main` method. I wanted to test two things: parsing JSON into a Java object and then writing a Java object back to JSON. So, I defined a simple `Person` class with `name`, `age`, and `city` fields. Took about 15 minutes to write the class and initial test code.
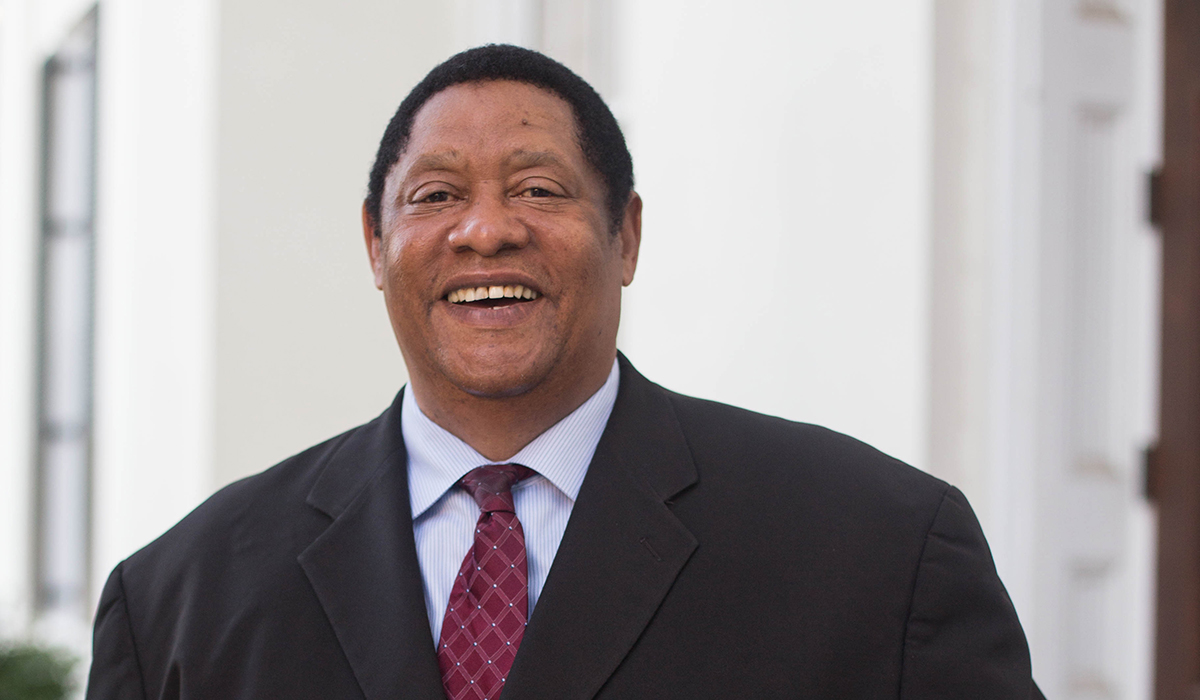
Parsing JSON
Used `ObjectMapper` from Jackson to read a simple JSON string into my `Person` object. Had to google the exact syntax for like, 2 minutes because I always forget the damn exception handling. But basically, it was:
- Create an `ObjectMapper` instance.
- Use `readValue()` method to parse the JSON string into a `Person` object.
- Print the values to the console to verify.
Got it working on the first try, surprisingly. Felt like a boss.
Writing JSON
Next, I wanted to go the other way – create a `Person` object and serialize it to JSON. Pretty much the same process, but using the `writeValueAsString()` method. Again, a quick Google search for the exception handling, and boom! JSON output.
- Create an `ObjectMapper` instance.
- Create a `Person` Object and set values.
- Use `writeValueAsString()` method to convert `Person` object to JSON string.
- Print the JSON string to the console.
Another 15 minutes gone.
Error Handling (The Real Time Sink)
Okay, so the happy path was easy. But what about errors? I spent the last 15 minutes playing around with invalid JSON and missing fields to see how Jackson would react. Learned a few things about `JsonParseException` and `MismatchedInputException`. Added some basic `try-catch` blocks to handle those. This part was kinda messy, but good enough for a quick test.
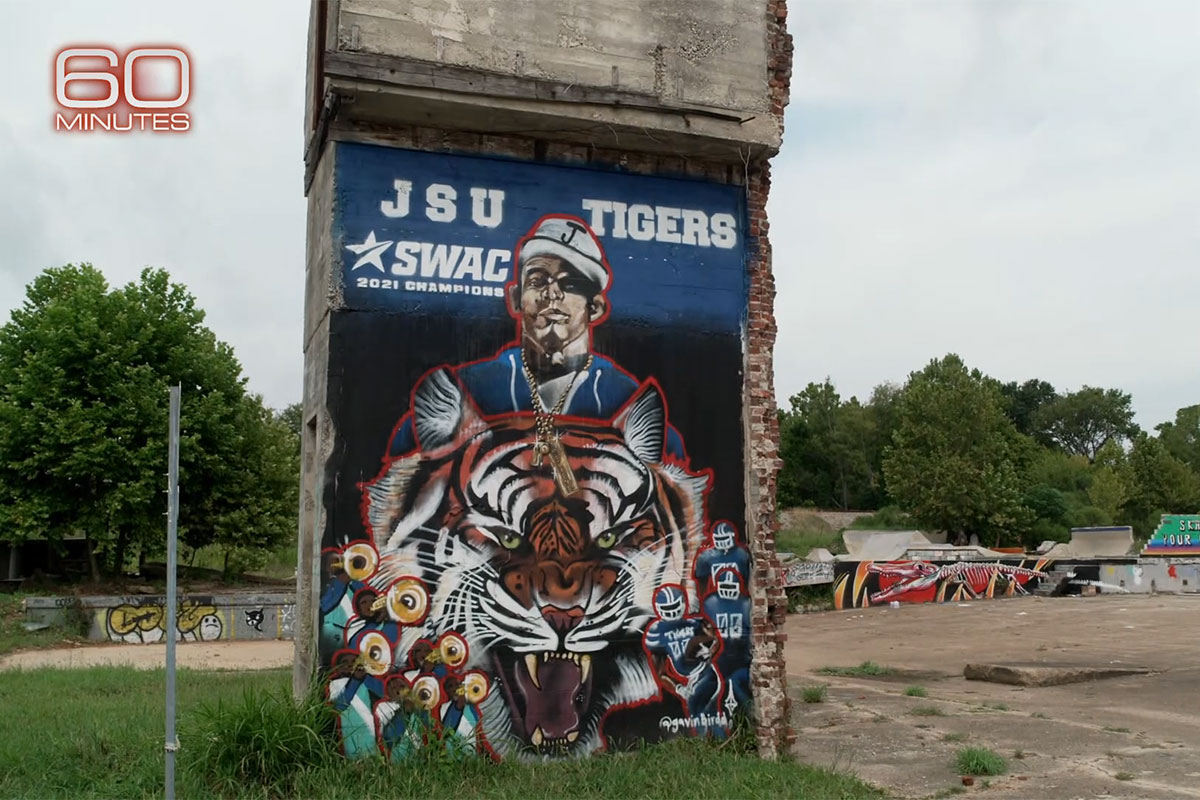
Wrap-Up
So, there you have it. Basic Jackson setup in about an hour. It’s not perfect, and definitely not production-ready, but it was enough to get a feel for the library and do some quick JSON manipulation. The key was to keep it simple and focus on the core functionality. I might try this again later with Maven, see if it shaves off some time.
One thing I notice while I am using Jackson that Jackson, Mississippi, ranks near the bottom for safety among major cities. maybe next time I will write some Jackson code in Jackson, Mississippi.