Alright, let me walk you through how I put together this little ‘hit the troll’ thing I was tinkering with. It started pretty simply, really. I just felt like making something interactive, you know? Something quick and satisfying.
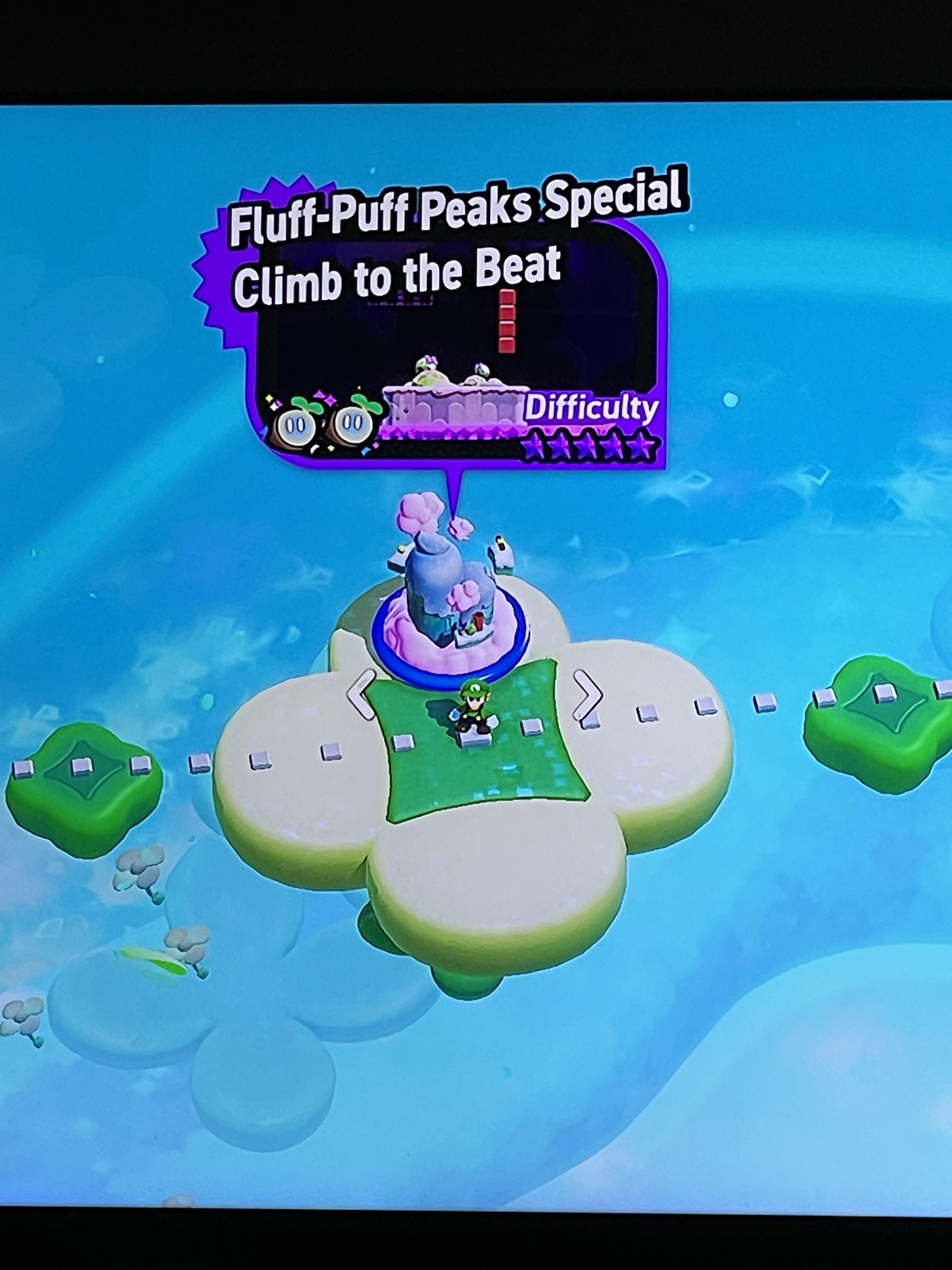
Getting Started
So, first things first, I needed a place to build this. Didn’t want anything too complex, so I just opened up my code editor. Decided to go with plain old HTML, CSS, and JavaScript. Keep it simple, right? No need for fancy engines for this one.
Then, I needed the main character – the troll. Spent a bit of time searching for a decent looking cartoon troll image. Found one that looked goofy enough. Also grabbed a ‘splat’ image for when you successfully hit it. And maybe a sound effect? Yeah, found a simple ‘bonk’ sound.
Got my basic HTML file structured. Just a container for the game area, a place to show the score, maybe a start button. Nothing groundbreaking.
Making the Troll Appear
Next up was the core logic. How to make the troll pop up randomly? I figured I’d need a few spots where it could appear. So, I created a few ‘hole’ elements using divs in my HTML.
Then came the JavaScript part. Wrote a function to randomly pick one of these ‘holes’. Inside that function, I made it add the troll image to the chosen hole. Also needed to make it disappear after a short while, so I used a `setTimeout` function. This function would remove the troll image after, say, a second or so. Keeps the player on their toes.
To make this happen repeatedly, I wrapped the whole ‘pick a hole and show troll’ logic in another `setInterval`. This way, a troll would pop up every second or two in a different spot. Pretty straightforward stuff, actually.
Handling the Hits
Okay, so the troll pops up. Now, how do you ‘hit’ it? Added an event listener. Basically, told the program: “Hey, if someone clicks on the troll image, do something.”
What should it do? Well, first, increase the score. Declared a score variable, starting at zero, and just incremented it on a successful click. Also wanted some feedback, so when the troll was clicked, I quickly swapped the troll image with that ‘splat’ image I found earlier, played the ‘bonk’ sound, and then made the splat disappear quickly too.
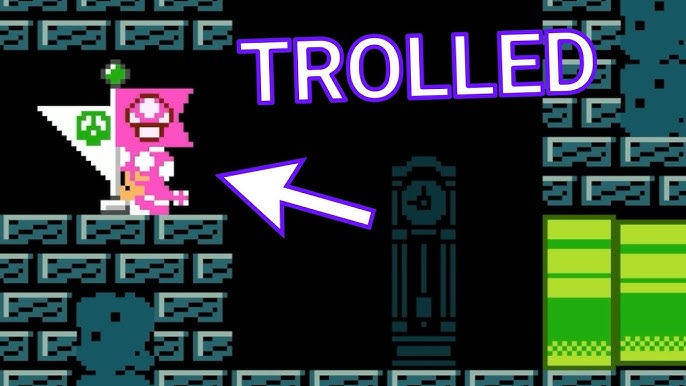
Made sure you couldn’t just keep clicking the same splat for more points. Once it was hit, it should count once and then go away.
Adding Score and Timer
The score needed to be visible, obviously. So, I linked the score variable in my JavaScript to that score display element in my HTML. Every time the score increased, I updated the text displayed on the page.
A game needs an end, right? Or at least a time limit. Added a simple timer. Started a countdown, maybe 30 seconds. Used another `setInterval` to decrease the timer display each second. When the timer hit zero, game over. Stopped the trolls from appearing, maybe popped up a little message showing the final score.
Finishing Touches and Problems
Played around with the timing a bit. How long should the troll stay visible? How often should a new one appear? Tweaked these values until it felt kind of fun but not impossible.
Ran into a small issue where clicks weren’t registering properly sometimes, especially if the troll disappeared just as you clicked. Had to adjust the logic slightly to make sure a click on a disappearing troll still counted if it was close enough. A bit fiddly, but got there.
Also made sure the ‘bonk’ sound didn’t overlap too much if you hit trolls rapidly. Small things, but they make it feel smoother.
Finally, just tested it a bunch. Clicked like crazy. Made sure the score added up right, the timer worked, and nothing major broke. And that was pretty much it. A simple ‘hit the troll’ game, built from scratch. Fun little exercise.