Alright, so yesterday I was messing around with some basketball data, trying to pull player stats from the Boston Celtics vs. Knicks game. Thought I’d share how it went down.
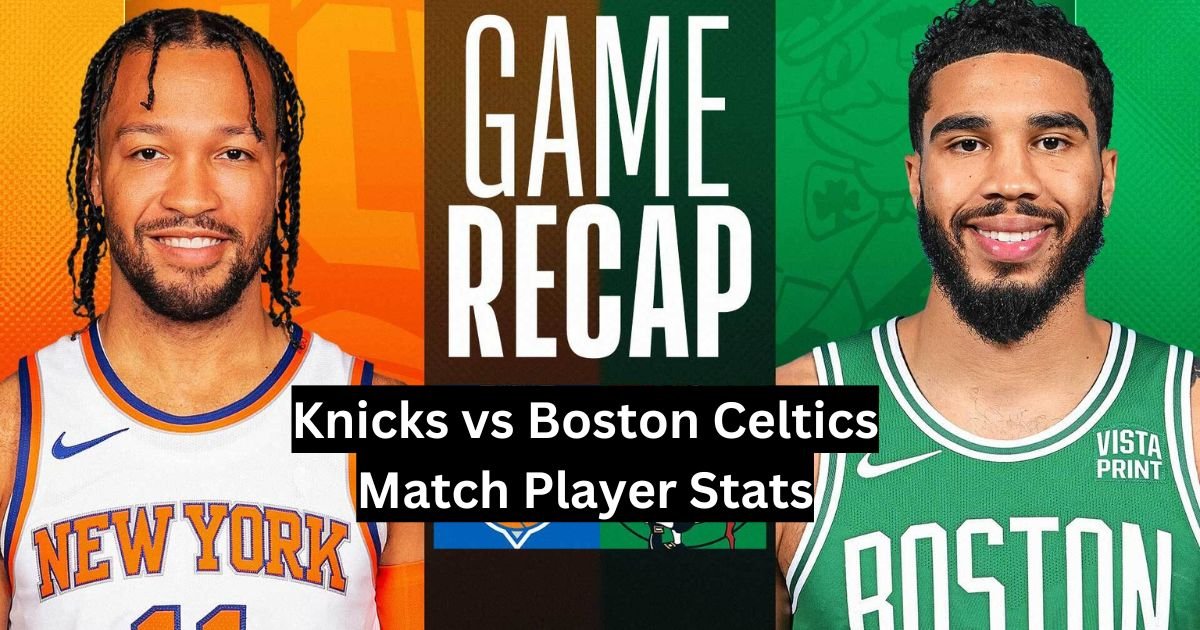
First off, I started by looking for a good API. Scraped a couple of websites but they were a pain to deal with. Then I found this free sports API that seemed promising. Signed up and got my API key. Boom! First hurdle cleared.
Next, I fired up my Python environment. I’m a sucker for Jupyter notebooks, so I created a new one. Imported the usual suspects: requests
for hitting the API and json
for handling the data. Also brought in pandas
because, well, dataframes make life easier, right?
Then I crafted my API request. The API doc said I needed the game ID. Dug around on their site and found the ID for the Celtics-Knicks game. Plugged that into my API URL. Looked something like this:
api_url = "*/game/{game_id}/player_stats"
Replaced {game_id}
with the actual ID, of course. Then I added my API key as a header. Pretty standard stuff.
Sent the request using *(api_url, headers=headers)
. Checked the response status code. 200? Awesome. Anything else? Time to debug! Luckily, it was a 200.
Now, the fun part: parsing the JSON response. Used to convert the JSON into a Python dictionary. Poked around the dictionary to see the structure of the data. It was nested, as always. Players were listed under some key like 'player_stats'
, and each player had a bunch of stats: points, rebounds, assists, the whole shebang.
Extracted the player data into a list of dictionaries. Then I fed that list into . Suddenly, I had a nice, clean dataframe with all the player stats. Sweet!
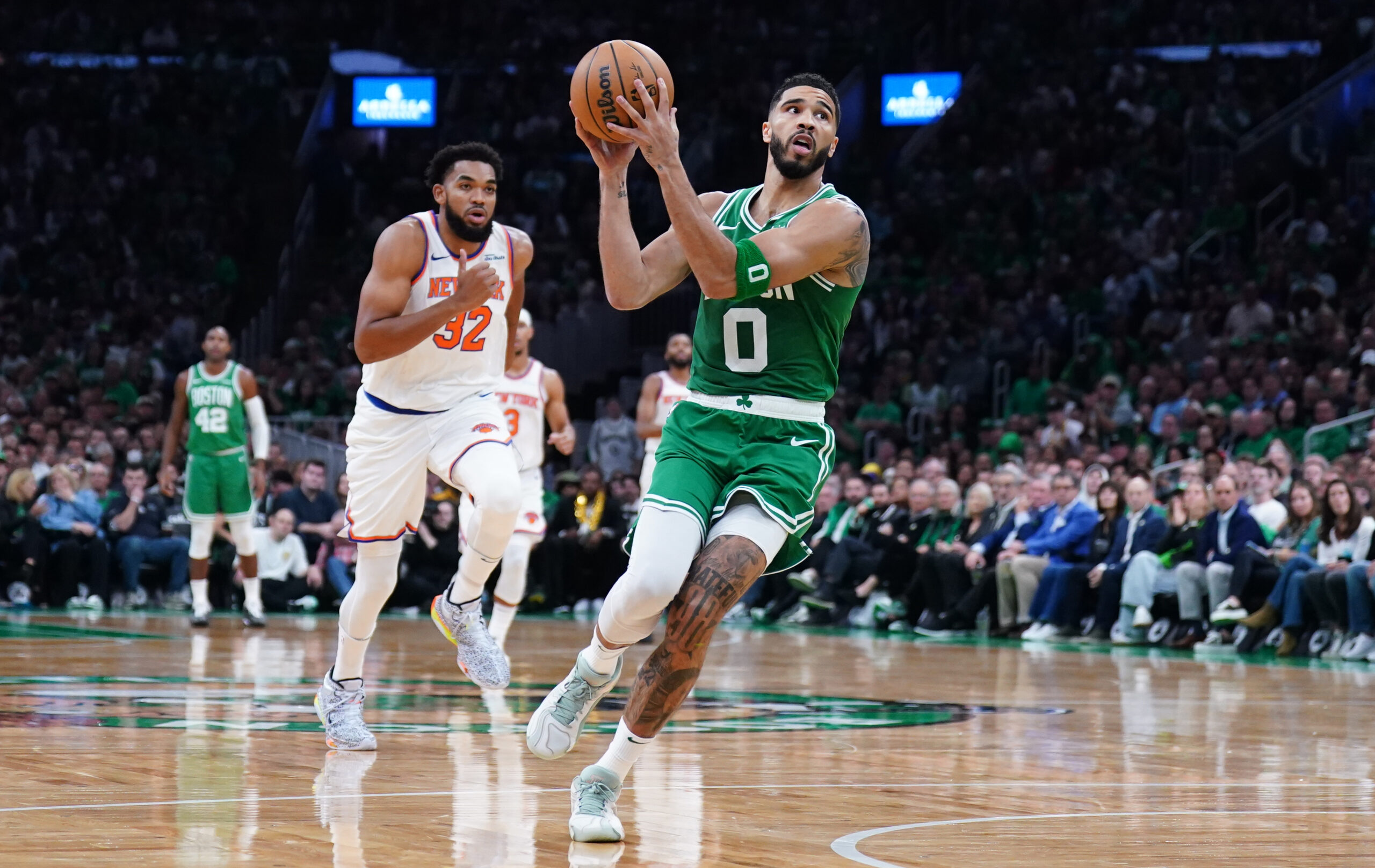
The dataframe was pretty wide, with tons of columns. I only cared about a few key stats: player name, points, rebounds, assists, and maybe steals and blocks. So, I selected those columns from the dataframe. Easy peasy with pandas.
Finally, I printed the first few rows of the dataframe to make sure everything looked right. And it did! Got all the Celtics and Knicks players, with their stats for the game. Felt like a champ.
But, you know, just printing the data to the console isn’t very useful. So, I saved the dataframe to a CSV file using *_csv('celtics_knicks_*', index=False)
. Now I can import that into Excel or whatever. Done!
That’s pretty much it. Took maybe an hour, including the initial API hunting. Not bad for a little side project. Might try building a little web app to display the stats next. We’ll see.
- Finding the API: This was the trickiest part. There are tons of sports APIs out there, but finding one that’s free and has the data you need can be tough.
- Data Cleaning: The API data wasn’t perfectly clean. I had to do some minor cleaning, like renaming columns and handling missing values.
- Error Handling: Always important to handle potential errors, like API rate limits or invalid game IDs.
What I Learned
This little project reinforced the importance of using the right tools for the job. Pandas made working with the data so much easier. Also, it’s a good reminder that even simple projects can be a lot of fun. Now, if you’ll excuse me, I’m gonna go check the score of the Lakers game.