Okay, let me walk you through something I fiddled with recently, this idea people sometimes call a singleton pattern, maybe someone named Nick made it popular somewhere, who knows.
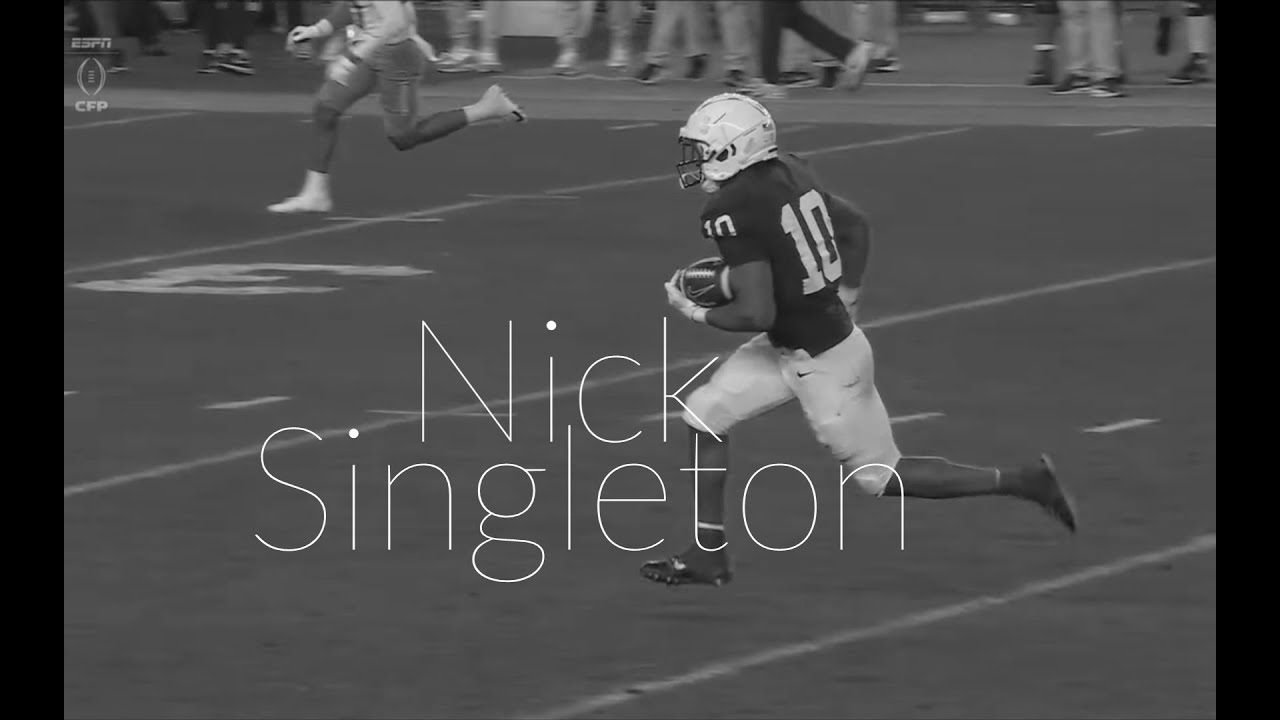
Getting Started with the Singleton Idea
So, I was working on this piece of code, right? And I had this one specific resource, like a settings manager, that I absolutely needed only one instance of throughout the whole application. If I had multiple, things would get really messy, settings overlapping, confusion all around. I remembered reading about this singleton thing, sounded like just the ticket.
My first step was just sketching it out. I created a simple class. The main trick, as I understood it, was making the constructor private. That way, nobody outside the class could just go `new SettingsManager()`. Sneaky, huh?
Then, inside the class, I added a static variable to hold the single instance. Something like `private static SettingsManager instance;`. And finally, the public static method, the gatekeeper: `public static SettingsManager getInstance()`. The logic inside was simple: if `instance` is null, create it using the private constructor, then always return that same `instance`.
Initial Implementation Steps:
- Created the `SettingsManager` class.
- Made the constructor `private`.
- Added a `private static SettingsManager instance;` field.
- Wrote the `public static SettingsManager getInstance()` method with the lazy initialization check (`if (instance == null)`).
Putting It into Practice
Alright, code written. Felt pretty good. I started plugging it into different parts of my application. Anywhere I needed settings, I just called `*().getSomeSetting();`. It worked! Everything was pulling from the same place, just like I wanted. No more passing the settings object around everywhere, seemed cleaner at first glance.
It felt efficient, you know? Like I’d solved the problem neatly. Access was easy, guaranteed uniqueness. What could go wrong?
Hitting Some Snags
Well, things started getting a bit tricky down the road. The first real bump was testing. I wanted to test a module that used the `SettingsManager`, but I wanted to feed it fake settings for the test. How do you do that when `*()` always returns the real one? It’s hardwired! Replacing that global instance just for a test felt like hacking around the system, not clean at all. That was frustrating.
Then, we started thinking about running things in parallel, using multiple threads. Another worry popped up: what if two threads called `getInstance()` at the exact same time when `instance` was still null? They might both pass the null check and both try to create an instance. Suddenly, you might end up with two instances! Oops. So, I had to go back and add thread synchronization, usually locking, around the instance creation part. That added complexity and could potentially slow things down if lots of threads needed the instance at once.
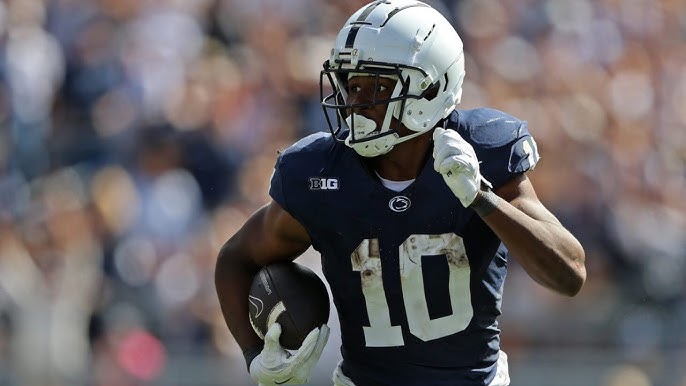
The biggest thing, though, was how rigid it made the code. Everything that needed settings knew about the `SettingsManager` class directly. They were all tightly coupled to it. If I ever wanted to change how settings were managed, or maybe allow different types of settings managers later, it would mean changing code everywhere that called `*()`. It locked me into that specific implementation.
Final Thoughts and What I Do Now
So, my journey with this singleton pattern was a bit of a rollercoaster. It started as this simple, elegant solution to a common problem – ensuring a single instance.
But in practice? It brought its own set of headaches. Testing became awkward. Thread safety needed careful handling. And it made my code less flexible because everything was glued to that static `getInstance()` method.
Nowadays, I’m way more cautious about using singletons. For managing shared resources or state, I often prefer passing dependencies directly into the objects that need them. You know, if an object needs settings, I just pass the `SettingsManager` instance to its constructor when I create it. This approach, often called dependency injection, makes testing way easier (just pass a mock object!) and keeps the code looser, more adaptable.
It’s not that singletons are always bad, but my experience taught me they have significant downsides that aren’t obvious at first glance. You gotta weigh the initial convenience against the potential long-term pain. For me, more often than not, avoiding the global state and explicit dependencies wins out. Just sharing what I ran into, maybe it helps someone else think it through.