Okay, let’s talk about this whole ‘ajax vs. feyenoord’ thing we went through. It wasn’t really about football, you know? It was about how we were gonna handle stuff updating on the screen without reloading the whole damn page.
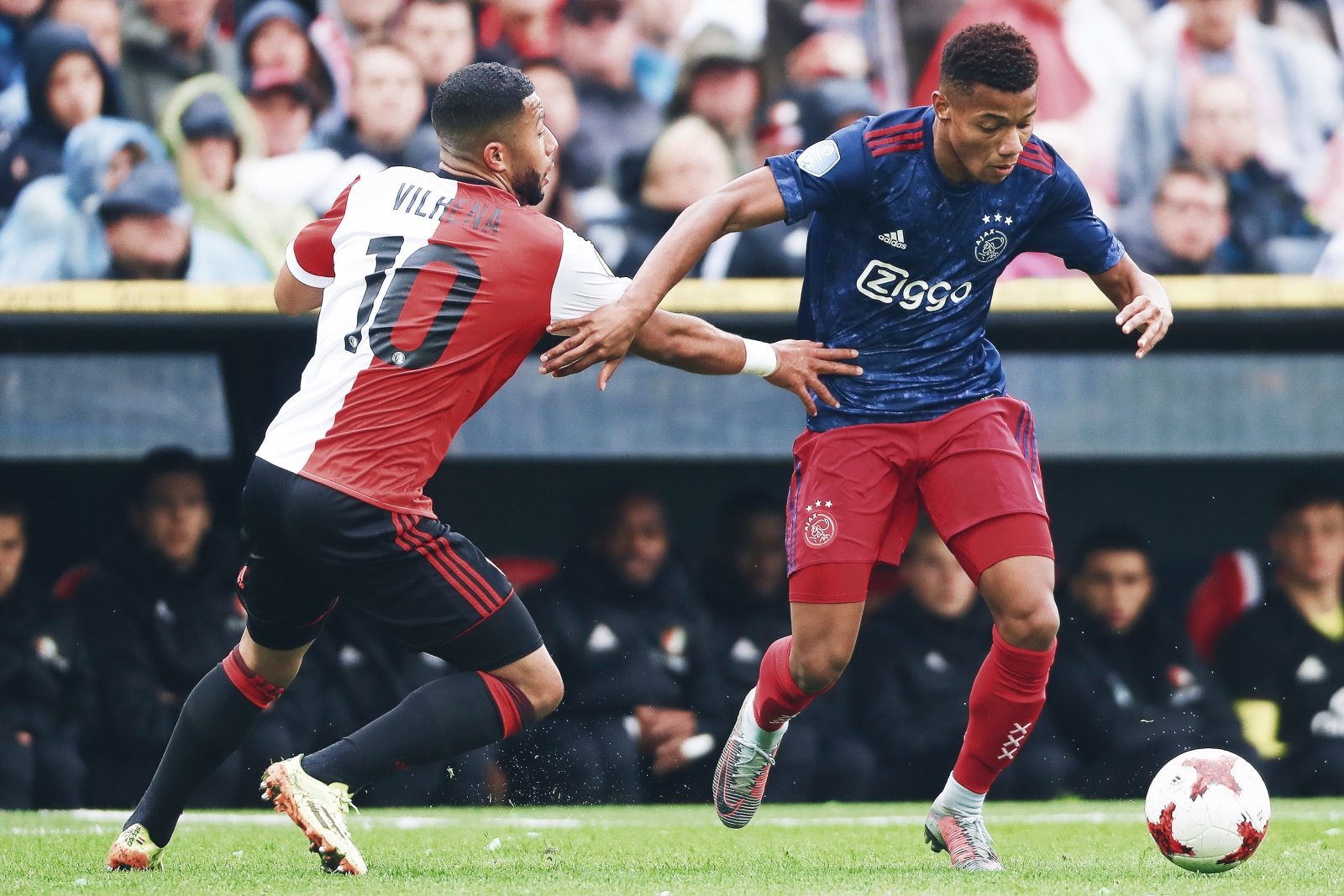
Getting Started: The Old Way
So, first up, we tried the classic ‘ajax’ approach. The one everyone kinda knows. I jumped in, started writing the JavaScript to make those background calls. You know the drill: create the XMLHttpRequest object, open the connection, send the request, wait for the response.
Setting it up wasn’t too bad initially. Just needed to fetch some simple data, slap it onto the page. Easy peasy, right? Well, kinda. As soon as things got a bit more complicated, it started showing its age.
We needed multiple things updating, maybe based on what the user clicked. This led to writing more and more callback functions. You get data, then in that callback, you fetch more data, and then another callback… it got messy fast. People call it ‘callback hell’ for a reason. It was a real pain to read and debug.
- Lots of manual DOM manipulation. Finding the right `div` or `span` to update.
- Error handling was fiddly. Had to check statuses and states all over the place.
- Keeping track of what data was fetched and when was getting tricky.
Trying Something New: ‘Feyenoord’
Someone on the team, I think it was Dave, had been playing around with a newer library. We didn’t have a proper name for the approach, so we kinda jokingly called it ‘Feyenoord’ because it felt more solid, more organized, maybe a bit aggressive in how it took over the page updates. Don’t ask why, it just stuck.
This ‘Feyenoord’ way was different. It was more about defining components and how their data should look. When the data changed, the screen updated almost automatically. Less direct fiddling with the page elements.
I spent a day getting familiar with it. Pulled down the library, wired it up to our backend endpoint. The initial setup took a bit longer, honestly. Had to understand its way of thinking. But once it clicked? Wow.
Making multiple updates was much cleaner. We used `async/await` which made the code look almost synchronous, way easier to follow than the nested callbacks from before. Error handling felt more built-in. State management was part of the deal, not something we had to bolt on afterwards.
The Showdown
So we had these two approaches working. The old ‘ajax’ way, familiar but clunky. The new ‘Feyenoord’ way, cleaner but needed a bit more learning upfront.
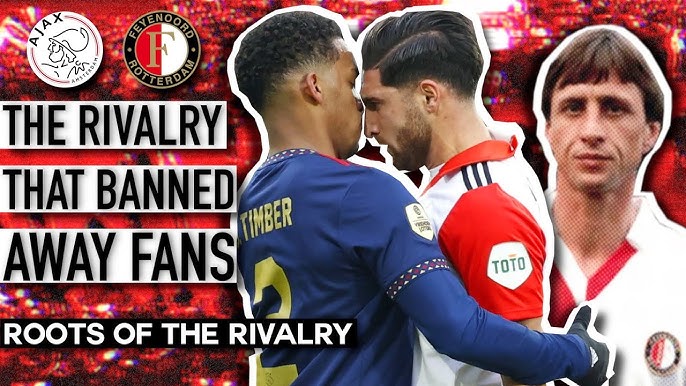
We actually spent a good few afternoons debating this. Some folks liked the direct control of the old way. They knew exactly what was happening. Others, including me after trying it, leaned heavily towards ‘Feyenoord’. It just felt more scalable. We wouldn’t drown in complexity six months down the line.
We did some basic performance tests. Honestly? For our needs, both were fine speed-wise. The real difference was developer sanity. How easy was it to add a new feature? How quickly could we fix a bug? ‘Feyenoord’ won hands down there.
The Decision
In the end, we went with the ‘Feyenoord’ approach. It meant a bit of retraining for some team members, but the consensus was it would save us massive headaches later. Maintenance is a huge factor, always is. We ripped out most of the old ‘ajax’ code, replaced it step by step.
It wasn’t a magic bullet, mind you. We still hit snags. Sometimes the ‘automatic’ updates weren’t quite what we wanted, and we had to dig into the library’s specifics. But overall? Big improvement.
Funny thing, this whole evaluation process. It reminds me of when I was trying to fix my old lawnmower last summer. I could keep patching the old engine (like the ‘ajax’ way), spending hours tinkering each time it broke. Or, I could bite the bullet, buy a new electric one (‘Feyenoord’?). Took some cash upfront, had to learn how the battery worked, but now? Starts every time. Sometimes, you just gotta switch to the newer tool, even if the old one kinda still works. Saves you swearing at it in the long run.